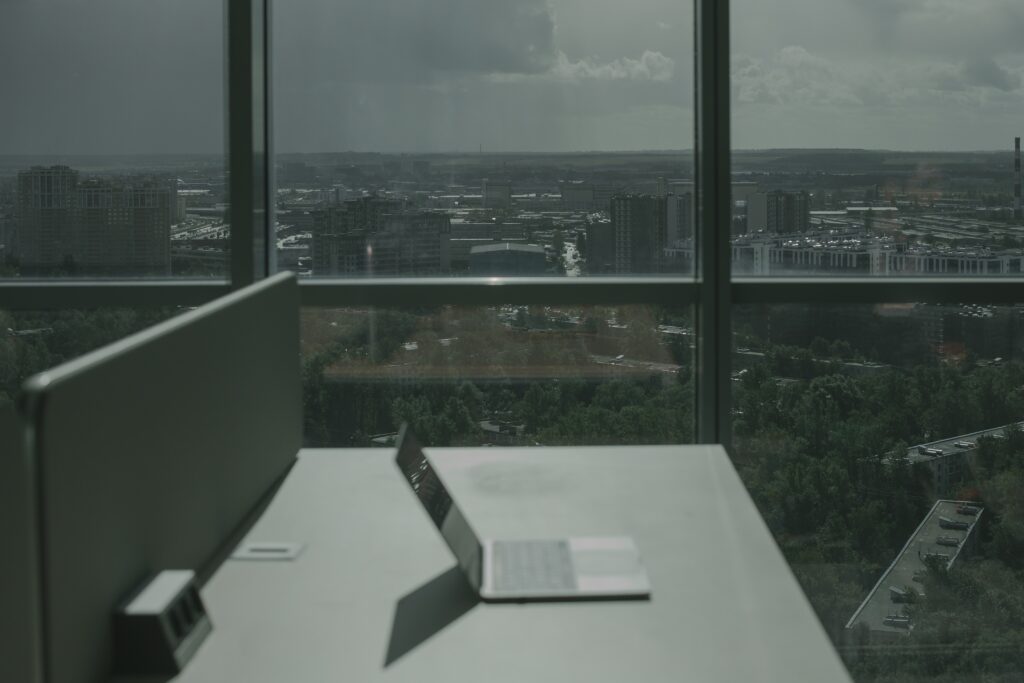
In the vast world of database management, SQL Inserts play a crucial role in adding data to tables. Whether you’re a seasoned developer or just starting to explore the realm of databases, understanding SQL Inserts is essential for efficient data manipulation. In this comprehensive guide, we will unravel the intricacies of SQL Inserts, exploring their syntax, advanced techniques, best practices, troubleshooting, and more. To learn more about SQL Language visit this blog post!
I. Introduction to SQL Inserts
SQL Inserts serve as the fundamental method for adding new records to a database table. By executing SQL Insert statements, you can seamlessly incorporate data into your tables, ensuring that your database remains up-to-date and accurate.
Within the realm of database management, SQL Inserts are indispensable when it comes to various scenarios, such as populating tables with initial data, importing data from external sources, or updating existing records.
A. Definition and Purpose of SQL Inserts
At its core, SQL Inserts involve adding new records or rows into a database table. This process is vital for populating tables with initial data, updating existing records, or importing data from external sources like CSV files or other databases. SQL Inserts provide the means to add data in a structured manner, ensuring that the database remains organized and consistent.
The purpose of SQL Inserts goes beyond simply adding data. It allows for data synchronization between different tables, databases, or even different systems, enabling seamless integration and data transfer. Whether you are building a web application, managing a large-scale enterprise database, or performing data analysis, SQL Inserts are essential for maintaining a well-functioning and up-to-date database.
B. Importance of SQL Inserts in Database Management
Database management involves not only creating tables and defining relationships but also handling the constant influx of new data. SQL Inserts provide the necessary tools to manage this data seamlessly. Without the ability to insert new records, databases would quickly become outdated and lose their utility.
SQL Inserts are particularly crucial in scenarios where real-time data is essential, such as e-commerce platforms, social media applications, or financial systems. By utilizing SQL Inserts, developers can ensure that newly generated information is promptly stored and made available for further processing and analysis.
C. Brief Overview of SQL Syntax and Structure
To effectively utilize SQL Inserts, it’s essential to have a basic understanding of SQL syntax and structure. SQL, or Structured Query Language, is a programming language designed specifically for managing relational databases. It provides a standardized way to interact with databases, including inserting, updating, querying, and deleting data.
SQL syntax follows a specific structure for each statement, including SQL Inserts. An SQL Insert statement consists of the INSERT INTO
clause, followed by the table name, column names (optional), and the corresponding values to be inserted. The structure of an SQL Insert statement allows for flexibility in specifying the exact values to be added to the database.
As we progress through this guide, we will explore the various components of SQL Insert statements in detail and provide practical examples to illustrate their usage.
II. Understanding SQL Insert Statements
To grasp the concept of SQL Inserts, it’s crucial to understand the syntax and structure of SQL Insert statements. At its core, an SQL Insert statement consists of the table name, column names, and the corresponding values to be inserted. By carefully crafting these statements, you can precisely control how data is added to your database.
In this section, we’ll delve into the components of SQL Insert statements, exploring how each element contributes to the overall insert process. Through examples and explanations, you’ll gain a solid foundation in constructing basic SQL Insert statements.
A. Syntax of SQL Insert Statements
The syntax of an SQL Insert statement follows a specific pattern. It starts with the INSERT INTO
clause, which indicates that new records will be added to a table. After the INSERT INTO
clause, the table name is specified, followed by optional column names and the corresponding values to be inserted.
Here’s a basic example of the syntax of an SQL Insert statement:
sql
INSERT INTO table_name (column1, column2, column3, ...)
VALUES (value1, value2, value3, ...);
In this example, table_name
represents the name of the table where the records will be inserted. The column1, column2, column3, ...
section is optional and allows you to specify the columns into which the values will be inserted. If the column names are omitted, the values will be inserted into all columns in the order they appear in the table’s schema.
The VALUES
keyword is followed by a list of values enclosed in parentheses. These values correspond to the columns specified in the previous section. The number of values must match the number of columns specified or the number of columns in the table if no column names are provided.
B. Components of SQL Insert Statements
To construct a valid SQL Insert statement, you need to understand the key components and their roles. Let’s explore each component in detail:
- Table Name: The table name is the destination where the new records will be inserted. It is essential to ensure that the table exists and that you have the necessary permissions to insert data into it.
- Column Names: The column names, although optional, allow you to specify the specific columns into which the values will be inserted. This gives you more control over the insertion process. If no column names are provided, the values will be inserted into all columns in the order they appear in the table’s schema.
- Values: The values represent the actual data that will be inserted into the specified columns. The number of values must match the number of columns specified or the number of columns in the table if no column names are provided. It’s essential to ensure that the data types of the values align with the corresponding columns in the table.
By understanding these components and their interactions, you can construct precise and accurate SQL Insert statements that meet your specific database requirements.
C. Examples of Basic SQL Insert Statements
To solidify our understanding of SQL Insert statements, let’s explore a few examples:
Example 1: Inserting a Single Record
Suppose we have a table named employees
with columns id
, name
, and position
. We want to insert a new employee with an ID of 1, a name of “John Doe,” and a position of “Software Engineer.” The corresponding SQL Insert statement would be:
sql
INSERT INTO employees (id, name, position)
VALUES (1, 'John Doe', 'Software Engineer');
This statement specifies the table name, column names, and the values to be inserted.
Example 2: Inserting Multiple Records
Sometimes, you might need to insert multiple records with a single SQL Insert statement. To achieve this, you can provide multiple sets of values within the same statement. Let’s consider an example where we want to add two employees to the employees
table:
sql
INSERT INTO employees (id, name, position)
VALUES (2, 'Jane Smith', 'Data Analyst'),
(3, 'Robert Johnson', 'Project Manager');
In this example, we’re inserting two records into the employees
table. Each set of values is enclosed in parentheses and separated by a comma.
By studying these examples and understanding the syntax and components of SQL Insert statements, you are well-equipped to start adding new records to your database tables.
III. Advanced Techniques for SQL Inserts
While basic SQL Insert statements provide a solid foundation, there are advanced techniques that can enhance your data manipulation capabilities. In this section, we’ll explore these techniques, enabling you to take your SQL Inserts to the next level.
One such technique involves inserting multiple rows with a single SQL Insert statement. By leveraging this approach, you can significantly improve the efficiency of your data insertion process. Additionally, we’ll delve into the concept of inserting data from one table into another, allowing you to seamlessly merge and update information across multiple tables. Furthermore, we’ll explore how subqueries can be utilized within SQL Insert statements, enabling you to perform complex data transformations during the insertion process.
A. Inserting Multiple Rows with a Single SQL Insert Statement
In certain situations, you may need to insert multiple rows into a table at once. Instead of executing separate Insert statements for each row, you can leverage the power of a single SQL Insert statement to insert multiple rows simultaneously. This approach not only saves execution time but also helps maintain the integrity and consistency of your data.
To insert multiple rows with a single SQL Insert statement, you can provide multiple sets of values within the VALUES
clause, separated by commas. Each set of values represents a row to be inserted. Let’s consider an example:
sql
INSERT INTO customers (id, name, email)
VALUES (1, 'John Doe', 'john.doe@example.com'),
(2, 'Jane Smith', 'jane.smith@example.com'),
(3, 'Robert Johnson', 'robert.johnson@example.com');
In this example, we’re inserting three rows into the customers
table. Each set of values represents the data for a single row, including the id
, name
, and email
columns. By providing multiple sets of values within the same Insert statement, we can efficiently insert multiple rows with minimal effort.
B. Inserting Data from One Table into Another
In addition to inserting data manually, SQL provides a powerful feature that allows you to insert data from one table directly into another. This technique is particularly useful when you need to transfer or merge data between tables within the same database or even across different databases.
To insert data from one table into another, you can utilize the INSERT INTO SELECT
statement. This statement allows you to specify the source table and the target table, along with any necessary conditions or transformations. Let’s explore an example:
sql
INSERT INTO target_table (column1, column2, column3, ...)
SELECT columnA, columnB, columnC, ...
FROM source_table
WHERE condition;
In this example, source_table
represents the table from which you want to extract and insert data, while target_table
is the destination table where the data will be inserted. The SELECT
statement specifies the columns to be selected from the source table, and any necessary conditions can be applied using the WHERE
clause.
By employing this technique, you can efficiently transfer data between tables, perform data transformations during the insertion process, and synchronize data across different database entities.
C. Using Subqueries in SQL Insert Statements
Subqueries are powerful tools that allow you to embed one query within another. They can be leveraged in SQL Insert statements to retrieve data from a source table and insert it into a target table based on specific conditions or criteria. By combining the capabilities of subqueries with SQL Inserts, you can perform complex data manipulations and insertions with ease.
Let’s consider an example where we want to insert data into a target table based on a condition specified in a subquery:
sql
INSERT INTO target_table (column1, column2, column3, ...)
SELECT columnA, columnB, columnC, ...
FROM source_table
WHERE columnX IN (SELECT columnY FROM another_table);
In this example, the subquery (SELECT columnY FROM another_table)
retrieves a set of values from another_table
. The outer query then uses these values to determine which rows from the source_table
should be inserted into the target_table
. By incorporating subqueries into SQL Insert statements, you can perform intricate data selections and insertions that align with your specific requirements.
By exploring these advanced techniques for SQL Inserts, you can elevate your data manipulation capabilities and efficiently handle complex scenarios. Whether you need to insert multiple rows, transfer data between tables, or utilize subqueries, these techniques will empower you to streamline your database operations and achieve optimal results.
IV. Best Practices for SQL Inserts
To ensure the integrity and optimal performance of your database, it’s crucial to follow best practices when working with SQL Inserts. In this section, we’ll discuss essential considerations and techniques that will empower you to handle SQL Inserts efficiently.
Data validation and sanitization play a critical role in maintaining data integrity. We’ll explore techniques for validating and sanitizing data before insertion, ensuring that only valid and clean data is added to your tables. Additionally, we’ll discuss handling duplicate records, as well as performance optimization techniques to enhance the speed and efficiency of your SQL Inserts. Lastly, we’ll delve into transaction management, emphasizing the importance of maintaining data consistency and integrity.
A. Data Validation and Sanitization
One of the crucial aspects of SQL Inserts is ensuring the validity and integrity of the data being inserted. Data validation involves checking the values against predefined rules or constraints to ensure they meet the expected criteria. Sanitization, on the other hand, involves removing any potentially harmful or unnecessary characters or data from the input.
By implementing data validation and sanitization, you can prevent data corruption, protect against SQL injection attacks, and maintain data consistency. Techniques such as input parameterization, data type validation, and length constraints can help ensure that only valid and safe data is inserted into your tables.
B. Handling Duplicate Records
Duplicate records can cause inconsistencies and confusion within your database. It’s essential to have strategies in place to handle duplicate data during SQL Inserts. Depending on your specific requirements, you can choose to ignore duplicates, update existing records, or reject the insertion altogether.
To handle duplicates, you can use techniques such as primary keys, unique constraints, or upsert operations (a combination of update and insert). Primary keys and unique constraints enforce uniqueness and prevent the insertion of duplicate records. Upsert operations allow you to update existing records if a duplicate is detected or insert a new record if it doesn’t already exist.
By implementing effective duplicate handling strategies, you can maintain data integrity and avoid unnecessary data redundancy.
C. Performance Optimization Techniques for SQL Inserts
Efficiently managing the performance of SQL Inserts is crucial, especially when dealing with large datasets or high-frequency insertions. Optimizing the performance of your SQL Inserts can enhance the overall responsiveness and scalability of your database.
Some performance optimization techniques include:
- Batch Inserts: Instead of executing individual Insert statements for each record, you can use batch inserts to insert multiple records in a single database transaction. This approach reduces the overhead of multiple round-trips to the database and can significantly improve performance.
- Index Management: Properly managing indexes on your database tables can enhance the performance of SQL Inserts. It’s important to strike a balance between the number and type of indexes to ensure efficient data insertion.
- Data Loading Tools: In some cases, when dealing with large datasets, using specialized data loading tools or utilities can significantly improve performance. These tools often provide optimized algorithms for bulk inserts and can handle large volumes of data more efficiently.
By employing these performance optimization techniques, you can ensure that your SQL Inserts are executed swiftly and efficiently, minimizing any potential bottlenecks and ensuring a smooth data insertion process.
D. Transaction Management
Transaction management plays a vital role in maintaining data consistency and integrity. By using transactions, you can group multiple SQL Inserts into a single logical unit, ensuring that either all the inserts succeed or none of them are applied. This atomicity property of transactions prevents partial or inconsistent data inserts.
By wrapping your SQL Inserts within a transaction, you can maintain data integrity, handle errors effectively, and provide a rollback mechanism in case of failures. It’s important to handle transaction boundaries appropriately, committing the transaction only when all the inserts within it are successful.
V. Troubleshooting and Common Mistakes with SQL Inserts
Even with a solid understanding of SQL Inserts, issues and mistakes can arise. In this section, we’ll equip you with troubleshooting techniques to tackle common challenges encountered during SQL Inserts.
We’ll explore common error messages and their meanings, helping you decipher and resolve issues quickly. Additionally, we’ll address constraints and data integrity problems that may arise during the insertion process. You’ll also gain insights into debugging SQL Insert statements effectively, enabling you to identify and rectify errors efficiently. Lastly, we’ll discuss prevention and recovery strategies for failed SQL Inserts, ensuring minimal disruption to your database operations.
A. Error Messages and their Meanings
When encountering errors during SQL Inserts, the database management system (DBMS) typically provides error messages that offer insights into the issue at hand. Understanding these error messages and their meanings can help you diagnose and resolve problems efficiently.
Some common error messages you may encounter during SQL Inserts include:
- Primary Key Violation: This error occurs when you attempt to insert a record with a primary key value that already exists in the table. To resolve this issue, you can either update the existing record or choose a different primary key value.
- Data Type Mismatch: This error occurs when the data type of the value you are trying to insert does not match the data type of the corresponding column in the table. Ensure that the data types align correctly to avoid this error.
- Constraint Violation: Constraints, such as unique constraints or foreign key constraints, can prevent the insertion of data that violates predefined rules. If you encounter a constraint violation error, review the constraint definition and ensure that the inserted data adheres to the specified constraints.
By carefully analyzing the error messages provided by the DBMS, you can pinpoint the issue and take appropriate actions to rectify it.
B. Handling Constraints and Data Integrity Issues
Constraints play a vital role in maintaining data integrity within your database. When working with SQL Inserts, it’s crucial to handle constraints effectively to prevent data inconsistencies and errors.
If you encounter constraint violations during SQL Inserts, there are a few strategies you can employ:
- Validate Data Before Insertion: Perform thorough data validation and sanitization before executing SQL Inserts. This ensures that the data being inserted adheres to the constraints defined on the table.
- Disable Constraints Temporarily: In some cases, you may need to temporarily disable constraints during the insertion process. This can be useful when inserting data into multiple related tables that have complex interdependencies. However, exercise caution when disabling constraints, as it can leave your database temporarily vulnerable to data inconsistencies.
- Handle Constraint Violations in Code: If a constraint violation occurs during an SQL Insert, you can catch the error in your application code and handle it appropriately. This might involve alerting the user, logging the error, or performing corrective actions.
By implementing these strategies, you can effectively handle constraints and maintain data integrity during SQL Inserts.
C. Debugging SQL Insert Statements
Debugging SQL Insert statements can be challenging, especially when dealing with complex queries or large datasets. However, there are techniques and tools available to help identify and resolve issues efficiently.
- Print and Review SQL Statements: Print the generated SQL Insert statements and review them for any syntax errors or unexpected values. This can help identify simple mistakes or inconsistencies.
- Use Logging and Error Handling: Implement robust logging and error handling mechanisms in your application code. These can provide valuable insights into the execution flow and help identify issues related to SQL Inserts.
- Break Down Complex Queries: If you are dealing with complex SQL Insert statements, break them down into smaller parts. Execute each part separately, verifying the results at each stage. This approach can help pinpoint the source of any issues.
- Utilize Database Debugging Tools: Many database management systems provide debugging tools that enable step-by-step execution of SQL statements. These tools allow you to track the execution flow, inspect variables, and identify any errors or unexpected behavior.
By employing these debugging techniques, you can effectively identify and rectify issues with your SQL Insert statements, ensuring their successful execution.
D. Prevention and Recovery from Failed SQL Inserts
Despite our best efforts, SQL Inserts can sometimes fail due to various factors, such as network issues, data inconsistencies, or unexpected errors. It’s essential to have strategies in place to prevent and recover from failed SQL Inserts.
- Transaction Rollback: When executing SQL Inserts within a transaction, you can employ a rollback mechanism to revert any changes made during a failed insertion. This ensures that the database remains in a consistent state.
- Error Handling and Notification: Implement error handling mechanisms in your application code to catch and handle errors gracefully. This can involve logging the error details, notifying the appropriate stakeholders, and taking necessary corrective actions.
- Data Backups: Regularly backup your database to minimize the impact of failed SQL Inserts. This allows you to restore the database to a previous state in the event of data loss or corruption.
By implementing preventive measures, employing robust error-handling strategies, and maintaining up-to-date backups, you can minimize the impact of failed SQL Inserts and ensure the integrity of your database.
VI. Conclusion
SQL Inserts are an essential aspect of database management, allowing you to add new records to your tables and keep your data up to date. In this comprehensive guide, we have explored the fundamentals of SQL Inserts, delving into their syntax, advanced techniques, best practices, troubleshooting, and more.
We began by understanding the definition and purpose of SQL Inserts, recognizing their significance in maintaining accurate and functional databases. We then dived into the syntax and structure of SQL Insert statements, exploring the components that make up these statements and how they contribute to the insertion process.
Moving on, we explored advanced techniques for SQL Inserts, including inserting multiple rows with a single statement, inserting data from one table into another, and utilizing subqueries to enhance data manipulation during insertions. These techniques provide you with the flexibility and efficiency needed to handle complex data scenarios.
To ensure the integrity and optimal performance of your SQL Inserts, we discussed best practices such as data validation and sanitization, handling duplicate records, optimizing performance, and transaction management. By following these best practices, you can maintain the quality and efficiency of your database operations.
We also examined the troubleshooting and common mistakes associated with SQL Inserts. Understanding error messages, handling constraints and data integrity issues, debugging SQL Insert statements, and having strategies in place for prevention and recovery from failed inserts are crucial skills for maintaining a robust database.
In conclusion, SQL Inserts are a fundamental tool in database management, empowering you to add, update, and merge records seamlessly. By mastering the art of SQL Inserts and applying the techniques and best practices outlined in this guide, you can unlock the full potential of your database management skills and ensure the accuracy and efficiency of your data operations.
Remember to continuously expand your knowledge and stay updated with the latest advancements and features in SQL Inserts, as database technologies evolve rapidly. With dedication and practice, you can become proficient in SQL Inserts and make significant contributions to the success of your projects and organizations.
Now that we have covered the key aspects of SQL Inserts, it’s time for you to apply this knowledge, experiment with different scenarios, and continue exploring the vast realm of database management.