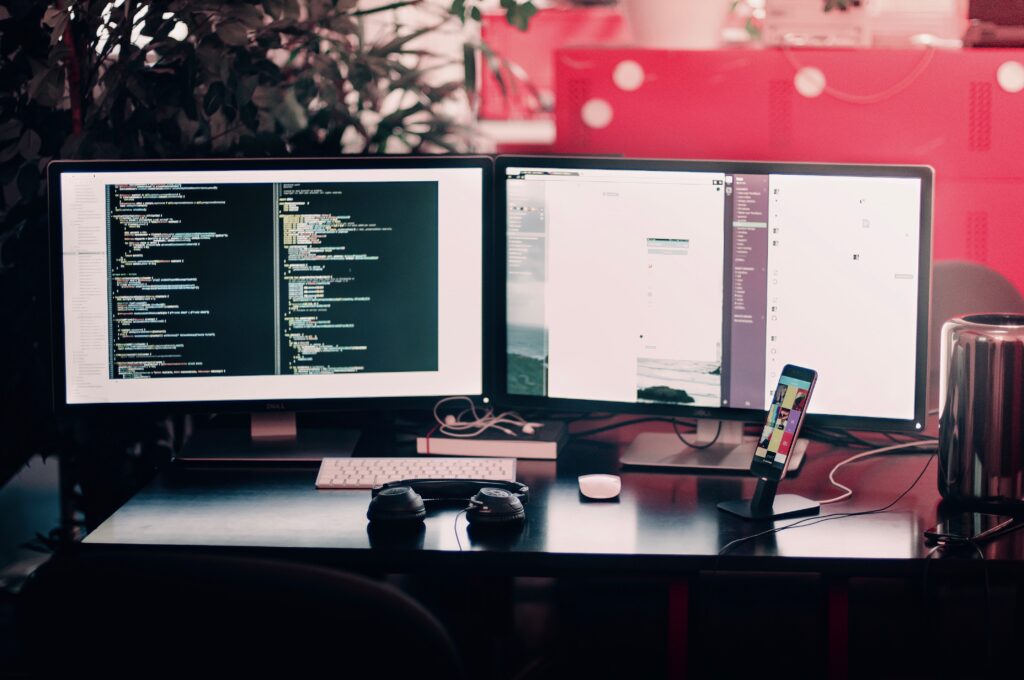
Structured Query Language, or SQL, is the backbone of most modern relational database management systems. It provides a standardized method to interact, manipulate, and retrieve data. Select commands in SQL allow users to extract precisely the data they need. In this extended guide, we’ll explore the depth and breadth of the SELECT
command, showcasing its versatility through examples.
1. The Essence of SELECT: The SELECT
command is primarily used to fetch data from a database. At its most basic, it can retrieve all columns from a specified table.
SELECT * FROM table_name;
For instance, to get all data from a table named Products
:
SELECT * FROM Products;
2. Handpicking Columns: More often than not, you might not need every column. Specify the ones you want for a more efficient query.
SELECT column1, column2, ... FROM table_name;
Example:
SELECT product_name, price FROM Products;
This fetches only the product names and their prices.
3. Filtering with WHERE: The WHERE
clause is a powerful tool that filters results based on conditions, making your data retrieval more precise.
SELECT column1, column2 FROM table_name WHERE condition;
Example:
SELECT product_name, price FROM Products WHERE price > 50;
This returns products priced above 50 units.
4. Sorting with ORDER BY: Organizing your results can be crucial for data analysis. The ORDER BY
clause sorts your results based on specified columns.
SELECT column1, column2 FROM table_name ORDER BY column_name [ASC|DESC];
Example:
SELECT product_name, price FROM Products ORDER BY price DESC;
This arranges products from the highest to the lowest price.
5. Limiting Data with LIMIT: Especially in large databases, you might want to limit the number of results. The LIMIT
clause helps you do just that.
SELECT column1, column2 FROM table_name LIMIT number;
Example:
SELECT product_name, price FROM Products LIMIT 5;
This fetches the first five products.
6. Aggregate Functions: SQL offers a suite of aggregate functions to perform calculations on your data, such as COUNT, SUM, AVG, MAX, and MIN. Example:
SELECT COUNT(product_name) FROM Products WHERE stock > 10;
This counts the products with a stock greater than 10.
7. Delving into JOINs: Data in relational databases is often distributed across multiple tables. The JOIN
clause is a robust tool that combines rows from two or more tables based on a related column. Example:
SELECT Orders.order_id, Customers.customer_name
FROM Orders
JOIN Customers
ON Orders.customer_id = Customers.id;
This fetches order IDs alongside the names of the customers who made them.
8. Grouping Data with GROUP BY: When using aggregate functions, you might want to group results based on certain columns. The GROUP BY
clause is perfect for this.
SELECT department, COUNT(employee_id)
FROM Employees
GROUP BY department;
This returns the number of employees in each department.
9. Having a Filter on Grouped Data: The HAVING
clause is used to filter results after a GROUP BY
operation.
SELECT department, COUNT(employee_id)
FROM Employees
GROUP BY department
HAVING COUNT(employee_id) > 10;
This fetches departments with more than 10 employees.
10. Combining Results with UNION: Sometimes, you might want to combine the results of two or more SELECT
statements. The UNION
operator does this.
SELECT product_name FROM Products
UNION
SELECT product_name FROM Archived_Products;
This returns product names from both current and archived products.
11. Nested Queries: Also known as subqueries, these are SELECT
statements within another SQL command. Example:
SELECT product_name
FROM Products
WHERE price > (SELECT AVG(price) FROM Products);
This fetches products priced above the average.
Conclusion: The SELECT commands in SQL are a testament to the language’s power and flexibility. From basic data retrieval to intricate operations involving multiple tables, aggregate functions, and nested queries, SELECT
offers a wide array of tools to extract the exact data you need. As with any tool, mastery comes with practice. By understanding and experimenting with the various clauses and functionalities associated with the SELECT
command, you can harness the full potential of your relational database.