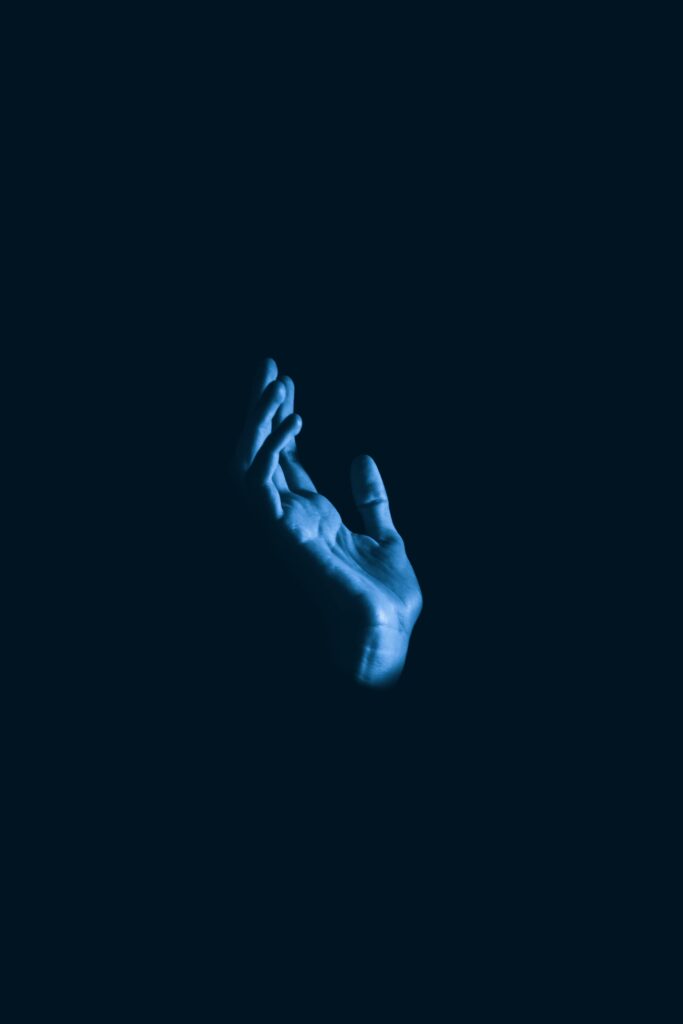
Have you ever found yourself struggling with complex SQL (Structured Query Language) queries, trying to make them more readable and efficient? If so, you’re in luck! In this comprehensive blog post, we will dive deep into the world of WITH AS in SQL, a powerful tool that can significantly enhance your SQL query capabilities.
Introduction to WITH AS in SQL
Before we delve into the intricacies of WITH AS in SQL, let’s start with a brief overview. WITH AS, also known as Common Table Expressions (CTEs), is a feature in SQL that allows you to define temporary result sets, which can then be referenced within the SQL query itself. This feature was introduced in SQL:1999 and has since become a staple in modern SQL databases.
Syntax and Usage of WITH AS in SQL
To harness the power of WITH AS in SQL, it’s essential to understand its syntax and usage. The WITH AS clause consists of one or more CTEs, each composed of a name (alias) and a query. These CTEs can be simple, recursive, or materialized, depending on your specific requirements. With this flexibility, you can tailor your CTEs to handle a wide range of scenarios.
Let’s take a closer look at each type of CTE:
- Simple CTEs: These are the most commonly used CTEs and provide a straightforward way to define a temporary result set. They are ideal for breaking down complex queries into manageable parts and improving code readability.
- Recursive CTEs: If you need to work with hierarchical or recursive data structures, recursive CTEs come to the rescue. They allow you to traverse and manipulate data in a recursive manner, making tasks like managing organizational charts or bills of materials a breeze.
- Materialized CTEs: Introduced in some databases as an extension to the standard CTE functionality, materialized CTEs enable the creation of temporary tables that store the result set of a CTE. This can significantly improve query performance by avoiding redundant computation.
Benefits and Advantages of WITH AS in SQL
Now that we understand the basics of WITH AS in SQL, let’s explore the benefits and advantages it offers. When utilized effectively, WITH AS can revolutionize your SQL querying experience in several ways:
Improved Readability and Maintainability of SQL Queries
One of the primary advantages of WITH AS is its ability to enhance the readability and maintainability of SQL queries. By breaking down complex queries into smaller, named CTEs, you can improve code organization and make your queries more understandable. This not only makes it easier for others to comprehend your code but also simplifies future modifications and troubleshooting.
Reducing Code Duplication and Enhancing Query Efficiency
WITH AS in SQL enables you to define a temporary result set once and reference it multiple times within a query. This eliminates the need to duplicate code or subqueries, resulting in cleaner and more efficient queries. Additionally, by precomputing result sets in CTEs, you can reduce the overall execution time and optimize query performance.
Simplifying Complex Queries and Enhancing Query Performance
Complex queries often involve multiple joins, subqueries, and calculations. WITH AS provides a powerful mechanism to simplify such queries by breaking them down into smaller, manageable parts. By dividing a complex problem into smaller subproblems, you can tackle each piece independently, greatly improving query comprehension and execution.
Enabling Recursive Queries and Hierarchical Data Manipulation
Manipulating hierarchical data structures, such as organizational charts or bill of materials, can be challenging in SQL. However, recursive CTEs empower you to traverse and manipulate hierarchical data using a recursive approach. This allows you to perform tasks like querying all descendants of a node or determining the depth of a hierarchy with ease.
Facilitating Data Transformation and Preparation for Analysis
Data transformation and preparation are critical steps in the data analysis process. WITH AS in SQL provides a convenient way to transform raw data into a format suitable for analysis. By applying various CTEs to filter, aggregate, or reshape data, you can streamline the data preparation process and generate meaningful insights more efficiently.
In the next section, we will explore the syntax and usage of WITH AS in SQL in more detail, providing examples to illustrate its practical implementation. Stay tuned to unlock the full potential of WITH AS in SQL!
Syntax and Usage of WITH AS in SQL
Now that we have a basic understanding of WITH AS in SQL, let’s dive deeper into its syntax and usage. This section will explore the structure and components of the WITH AS clause and provide examples to illustrate its practical implementation.
Understanding the Structure and Components of the WITH AS Clause
The WITH AS clause consists of one or more CTEs, each composed of a name (alias) and a query. The structure of a WITH AS clause can be summarized as follows:
WITH cte_name AS (
SELECT column1, column2, ...
FROM table_name
WHERE conditions
)
Here, cte_name
represents the name or alias given to the CTE, which can be used to reference the result set within the SQL query. The SELECT statement within the CTE defines the columns to be included in the result set, and the FROM clause specifies the table(s) from which the data is retrieved. Additionally, you can include WHERE conditions to filter the data as needed.
Exploring the Different Types of Common Table Expressions (CTEs)
WITH AS in SQL allows for the creation of different types of CTEs, each serving a specific purpose. Let’s take a closer look at the three main types of CTEs:
1. Simple CTEs
Simple CTEs are the most commonly used type of CTEs. They provide a straightforward way to define a temporary result set that can be referenced within the main query. Simple CTEs are ideal for breaking down complex queries into manageable parts, improving code readability, and making the query logic more apparent.
Here’s an example of a simple CTE in action:
sql
WITH sales_cte AS (
SELECT product_name, SUM(quantity) AS total_quantity
FROM sales
GROUP BY product_name
)
SELECT product_name, total_quantity
FROM sales_cte
WHERE total_quantity > 100;
In this example, the CTE named sales_cte
calculates the total quantity of each product from the sales
table using the SUM
function and GROUP BY
clause. The main query then selects the product names and total quantities from the CTE, filtering the result to only include products with a total quantity greater than 100.
2. Recursive CTEs
Recursive CTEs are designed to handle hierarchical or recursive data structures. They enable the traversal and manipulation of data in a recursive manner, making tasks like managing organizational charts or bills of materials more manageable. Recursive CTEs are especially useful when dealing with self-referential tables, where a record can have a relationship with another record within the same table.
A classic example of a recursive CTE is calculating the sum of all numbers from 1 to a given number. Let’s see how this can be achieved using a recursive CTE:
sql
WITH RECURSIVE numbers_cte (n) AS (
SELECT 1
UNION ALL
SELECT n + 1
FROM numbers_cte
WHERE n < 10
)
SELECT SUM(n) AS total_sum
FROM numbers_cte;
In this example, the CTE named numbers_cte
starts with an initial value of 1 and recursively adds 1 to the previous value until it reaches the condition n < 10
. The main query then calculates the sum of all the numbers generated by the recursive CTE, resulting in a total sum of 55.
3. Materialized CTEs
Materialized CTEs, available in some databases as an extension to the standard CTE functionality, allow you to create temporary tables that store the result set of a CTE. This can significantly improve query performance by avoiding redundant computation. Materialized CTEs work by materializing the result set into a temporary table, which can then be referenced multiple times within the query.
While the syntax and usage of materialized CTEs may vary depending on the database system, they generally involve the creation of a temporary table and populating it with the result set of the CTE. The temporary table can then be queried just like any other table, providing faster access to the pre-computed data.
Examples of WITH AS Syntax in SQL Queries
To solidify our understanding of the WITH AS syntax, let’s explore a few examples that demonstrate its practical usage in SQL queries.
Example 1: Calculating Employee Salaries
Suppose we have a database table called employees
that stores information about employees, including their salaries. We can use a simple CTE to calculate the total salary for all employees:
sql
WITH salary_total AS (
SELECT SUM(salary) AS total_salary
FROM employees
)
SELECT total_salary
FROM salary_total;
In this example, the CTE named salary_total
calculates the sum of all salaries from the employees
table. The main query then selects the total salary from the CTE, providing the result of the salary calculation.
Example 2: Recursive CTE for Organizational Chart
Consider a scenario where we have an organizational chart stored in a database table called employees
, with each record representing an employee and their direct manager. We can use a recursive CTE to traverse the organizational hierarchy and retrieve information about each employee and their respective manager:
sql
WITH RECURSIVE org_chart AS (
SELECT employee_id, employee_name, manager_id, 0 AS level
FROM employees
WHERE manager_id IS NULL
UNION ALL
SELECT e.employee_id, e.employee_name, e.manager_id, o.level + 1
FROM employees e
INNER JOIN org_chart o ON e.manager_id = o.employee_id
)
SELECT employee_id, employee_name, manager_id, level
FROM org_chart;
In this example, the recursive CTE named org_chart
starts with the top-level employees (those with manager_id
as NULL) and recursively joins with their respective subordinates. The result is a hierarchical representation of the organizational chart, including the employee ID, name, manager ID, and the level of the employee within the hierarchy.
These examples provide a glimpse into the versatility and power of WITH AS in SQL. By properly utilizing the WITH AS clause with different types of CTEs, you can tackle a wide range of data manipulation and analysis tasks more efficiently.
Benefits and Advantages of WITH AS in SQL
Now that we have explored the syntax and usage of WITH AS in SQL, let’s delve into the numerous benefits and advantages it offers. By harnessing the power of WITH AS, you can revolutionize your SQL querying experience and elevate the efficiency and effectiveness of your data manipulation tasks. Let’s dive into the key advantages of using WITH AS in SQL.
Improved Readability and Maintainability of SQL Queries
One of the primary benefits of using WITH AS in SQL is the improved readability and maintainability of your queries. By breaking down complex queries into smaller, named CTEs, you can enhance code organization and make your queries more understandable. This not only makes it easier for others to comprehend your code but also simplifies future modifications and troubleshooting.
Consider a scenario where you have a SQL query with multiple subqueries and joins. Without WITH AS, the query can quickly become convoluted and challenging to decipher. However, by using WITH AS to define meaningful CTEs for each subquery, you can separate the logic into more manageable parts. This enhances the readability of the query, making it easier to understand the intention and flow of the code.
Furthermore, the use of descriptive names for CTEs can provide a clear representation of the purpose of each subquery. This clarity in naming conventions allows developers to quickly grasp the intent of the query and facilitates collaboration among team members. By improving the readability and maintainability of your SQL queries, WITH AS empowers you to write cleaner and more organized code.
Reducing Code Duplication and Enhancing Query Efficiency
Another significant advantage of WITH AS in SQL is its ability to reduce code duplication and enhance query efficiency. With WITH AS, you can define a temporary result set once and reference it multiple times within a query. This eliminates the need to repeatedly write lengthy subqueries or duplicate code segments, resulting in cleaner and more efficient queries.
Consider a scenario where you need to calculate different aggregates based on the same subset of data. Without WITH AS, you may need to repeat the subquery multiple times within your main query. This not only increases the query’s complexity but also hampers its maintainability. By leveraging WITH AS, you can define the subquery once as a CTE and reference it multiple times within the main query, reducing code duplication and improving query efficiency.
Additionally, WITH AS can enhance query performance by optimizing the execution plan. Since CTEs are evaluated only once, the database optimizer can leverage this knowledge to generate a more efficient execution plan. By reducing redundant computations and streamlining the query execution process, WITH AS can significantly improve the overall performance of your SQL queries.
Simplifying Complex Queries and Enhancing Query Performance
Complex queries often involve multiple joins, subqueries, and calculations. WITH AS in SQL provides a powerful mechanism to simplify such queries by breaking them down into smaller, manageable parts. By dividing a complex problem into smaller subproblems, you can tackle each piece independently, greatly improving query comprehension and execution.
Let’s consider a scenario where you need to retrieve data from multiple tables and perform various calculations and aggregations. Without WITH AS, the query can quickly become convoluted and challenging to maintain. However, by utilizing WITH AS to define CTEs for each logical unit of the query, you can modularize the code and simplify the overall query structure.
For example, you can define separate CTEs for retrieving data from different tables, performing calculations, and aggregating results. By breaking down the complex query into smaller, self-contained parts, you can focus on addressing individual requirements more efficiently. This approach not only simplifies the query logic but also enhances query performance by allowing the database optimizer to optimize each CTE independently.
Enabling Recursive Queries and Hierarchical Data Manipulation
Managing hierarchical or recursive data structures can be challenging in SQL. However, WITH AS in SQL provides a powerful solution by enabling recursive queries. Recursive CTEs allow you to traverse and manipulate hierarchical data using a recursive approach, simplifying tasks such as managing organizational charts or bills of materials.
Consider an organizational chart where employees are represented in a self-referential table, with each record having a reference to its manager’s ID. By utilizing a recursive CTE, you can easily navigate the hierarchy and retrieve valuable information about each employee and their respective manager.
Recursive CTEs work by defining an initial anchor member and recursively joining with subsequent members until a termination condition is met. This recursive approach allows you to traverse the hierarchical data structure, perform calculations, and retrieve relevant information at each level of the hierarchy.
Facilitating Data Transformation and Preparation for Analysis
Data preparation and transformation are critical steps in the data analysis process. WITH AS in SQL provides a convenient way to transform raw data into a format suitable for analysis. By applying various CTEs to filter, aggregate, or reshape data, you can streamline the data preparation process and generate meaningful insights more efficiently.
For example, you may need to perform operations such as data cleansing, data aggregation, or data filtering before conducting analysis. Using WITH AS, you can define CTEs that encapsulate these data transformation operations, allowing you to focus on the specific requirements of each transformation step.
The flexibility of WITH AS allows you to chain multiple CTEs together, enabling a seamless flow of data transformation operations. This approach facilitates modularity and reusability, making it easier to modify or expand the data preparation process as needed.
In conclusion, WITH AS in SQL offers numerous benefits and advantages that empower you to write cleaner, more efficient, and more maintainable SQL queries. By improving readability, reducing code duplication, simplifying complex queries, enabling recursive queries, and facilitating data transformation, WITH AS unlocks the true potential of SQL and enhances your data manipulation capabilities.
Advanced Techniques and Best Practices for Using WITH AS in SQL
Now that we have explored the benefits and advantages of using WITH AS in SQL, let’s dive into some advanced techniques and best practices to maximize its potential. By leveraging these techniques, you can unlock additional functionalities and optimize the performance of your SQL queries. Let’s explore how to make the most out of WITH AS in SQL.
Utilizing WITH AS with Joins, Subqueries, and Aggregations
WITH AS in SQL is not limited to simple SELECT statements; it can be combined with joins, subqueries, and aggregations to solve more complex problems. By incorporating these techniques, you can leverage the power of WITH AS in a wide range of scenarios.
Joins with WITH AS
When working with multiple tables, joining them can provide a comprehensive view of the data. WITH AS allows you to define CTEs for each table and then join them together in the main query. This approach improves code organization and simplifies the query logic.
Consider a scenario where you have a customers
table and an orders
table, and you want to retrieve customer information along with their order details. You can define separate CTEs for each table and join them in the main query using a common key, like customer ID:
sql
WITH customers_cte AS (
SELECT customer_id, customer_name, email
FROM customers
),
orders_cte AS (
SELECT order_id, customer_id, order_date, total_amount
FROM orders
)
SELECT c.customer_id, c.customer_name, c.email, o.order_id, o.order_date, o.total_amount
FROM customers_cte c
JOIN orders_cte o ON c.customer_id = o.customer_id;
In this example, the CTEs customers_cte
and orders_cte
retrieve customer information and order details, respectively. The main query then joins these CTEs based on the customer ID to obtain the desired result set.
Subqueries within WITH AS
WITH AS can also be combined with subqueries to solve complex data retrieval problems. Subqueries can be used within CTEs to filter or transform data before including it in the final result set.
Consider a scenario where you want to retrieve all customers who have placed an order within the last month. You can use a subquery within a CTE to filter the customers based on the order dates:
sql
WITH recent_orders_cte AS (
SELECT customer_id
FROM orders
WHERE order_date >= CURRENT_DATE - INTERVAL '1 month'
GROUP BY customer_id
)
SELECT c.customer_id, c.customer_name, c.email
FROM customers c
JOIN recent_orders_cte r ON c.customer_id = r.customer_id;
In this example, the CTE recent_orders_cte
uses a subquery to retrieve the customer IDs of those who have placed an order within the last month. The main query then joins this CTE with the customers
table to obtain the relevant customer information.
Aggregations within WITH AS
WITH AS can also be combined with aggregations to perform calculations on subsets of data before including them in the final result set. This approach is particularly useful when you need to calculate summary statistics or perform complex calculations.
Consider a scenario where you want to calculate the total sales for each product category. You can use WITH AS to define a CTE that aggregates the sales data by product category and then use it in the main query:
sql
WITH sales_by_category_cte AS (
SELECT category, SUM(sales_amount) AS total_sales
FROM sales
GROUP BY category
)
SELECT category, total_sales
FROM sales_by_category_cte;
In this example, the CTE sales_by_category_cte
aggregates the sales data by product category, calculating the total sales amount for each category. The main query then selects the category and total sales from the CTE, providing the desired summary statistics.
By combining WITH AS with joins, subqueries, and aggregations, you can handle more complex data retrieval scenarios and perform advanced calculations with ease. This flexibility allows you to solve a wide range of problems, making your SQL queries more powerful and efficient.
Applying Filtering and Ordering within WITH AS Queries
WITH AS in SQL provides the flexibility to apply filtering and ordering within your CTEs. This allows you to refine the result set before referencing it in the main query, improving query performance and providing more control over the data.
Filtering within WITH AS
Filtering within WITH AS queries involves applying conditions to the CTE definition itself. This helps in reducing the amount of data processed in subsequent steps, leading to improved performance.
Consider a scenario where you only want to include customers who have placed more than five orders in a CTE. You can apply a filtering condition directly within the CTE definition:
sql
WITH frequent_customers_cte AS (
SELECT customer_id, COUNT(*) AS order_count
FROM orders
GROUP BY customer_id
HAVING COUNT(*) > 5
)
SELECT c.customer_id, c.customer_name, c.email
FROM customers c
JOIN frequent_customers_cte f ON c.customer_id = f.customer_id;
In this example, the CTE frequent_customers_cte
filters the orders by customer ID and counts the number of orders for each customer. The HAVING
clause ensures that only customers with more than five orders are included in the CTE. The main query then joins this CTE with the customers
table to obtain the desired result set.
Ordering within WITH AS
Ordering within WITH AS queries allows you to sort the result set of a CTE based on specific criteria. This can be useful when you want to control the order in which the data is presented in the main query.
Consider a scenario where you want to retrieve the top five customers with the highest total order amounts. You can use WITH AS to calculate the total order amounts for each customer and then order the result set within the CTE:
sql
WITH total_order_amounts_cte AS (
SELECT customer_id, SUM(order_amount) AS total_amount
FROM orders
GROUP BY customer_id
ORDER BY total_amount DESC
LIMIT 5
)
SELECT c.customer_id, c.customer_name, c.email, t.total_amount
FROM customers c
JOIN total_order_amounts_cte t ON c.customer_id = t.customer_id;
In this example, the CTE total_order_amounts_cte
calculates the total order amounts for each customer and orders the result set in descending order. The LIMIT
clause ensures that only the top five customers with the highest order amounts are included in the CTE. The main query then joins this CTE with the customers
table to obtain the desired result set.
By applying filtering and ordering within WITH AS queries, you can fine-tune the result set and improve the performance of your SQL queries. This level of control allows you to extract the most relevant and meaningful information from your data.
Optimizing Performance of WITH AS Queries
Optimizing the performance of WITH AS queries involves understanding the execution plans, employing indexing strategies, and analyzing query performance to identify and address potential bottlenecks. Let’s explore some techniques to optimize the performance of your WITH AS queries.
Understanding Execution Plans and Query Optimization Techniques
To optimize the performance of your WITH AS queries, it’s crucial to understand the execution plans generated by the database optimizer. Execution plans provide insights into how the database engine plans to execute the query and can help identify areas for optimization.
By examining the execution plans, you can identify potential performance bottlenecks such as unnecessary full table scans, inefficient join operations, or suboptimal index usage. Understanding the execution plans enables you to make informed decisions on how to optimize your queries.
To obtain the execution plan for a query, you can use the EXPLAIN statement. This statement provides a detailed breakdown of the steps the database engine will take to execute the query, including the order of operations, join strategies, and access methods.
Once you have the execution plan, you can analyze it to identify potential areas for optimization. Look for operations that involve large data sets, inefficient join algorithms, or missing or underutilized indexes. Based on this analysis, you can make informed decisions on how to optimize your WITH AS queries.
Indexing Strategies for WITH AS Queries
Indexes play a crucial role in optimizing query performance, including WITH AS queries. By carefully selecting and creating appropriate indexes, you can significantly improve the efficiency of your queries.
When using WITH AS queries, consider creating indexes on the columns used for joining, filtering, or ordering within the CTEs. These indexes can help reduce the execution time by allowing the database engine to quickly locate the relevant data.
For example, if you have a CTE that involves joining multiple tables on a specific column, consider creating an index on that column. This index can improve the join performance by allowing the database engine to efficiently locate the matching rows.
Similarly, if you have filtering conditions within a CTE, create indexes on the columns involved in those conditions. Indexes can significantly speed up the data retrieval process by enabling the database engine to quickly identify the relevant rows.
When it comes to ordering within WITH AS queries, indexes can also play a crucial role. By creating indexes on the columns used for ordering, you can avoid costly sorting operations and improve query performance.
However, keep in mind that creating too many indexes can also have a negative impact on performance, as they require additional disk space and may slow down data modification operations. It’s important to strike a balance between the number of indexes and the performance gains they provide.
Analyzing Query Performance and Identifying Bottlenecks
To optimize the performance of your WITH AS queries, it’s essential to analyze query performance and identify potential bottlenecks. By monitoring and measuring the execution time, resource consumption, and query statistics, you can gain insights into the areas that require optimization.
Database management systems provide various tools and techniques to analyze query performance. These tools can help you identify queries that consume excessive resources, perform poorly, or have long execution times. Some common techniques include query profiling, monitoring system resources, and analyzing query execution statistics.
By analyzing query performance, you can identify potential bottlenecks such as inefficient algorithms, suboptimal join strategies, or lack of appropriate indexes. Armed with this information, you can make informed decisions on how to optimize your WITH AS queries and improve overall system performance.
Optimizing the performance of WITH AS queries is an ongoing process. As data volumes grow and query complexity increases, it’s essential to continuously monitor and analyze query performance to ensure optimal execution times and resource utilization.
Handling Error and Exception Cases in WITH AS Queries
When working with WITH AS queries, it’s important to consider error and exception handling to ensure data integrity and prevent unexpected behavior. WITH AS queries can encounter various error scenarios, such as missing or invalid data, division by zero, or constraint violations. Proper error handling can help mitigate these issues and provide a robust and reliable query execution.
To handle errors and exceptions in WITH AS queries, consider the following best practices:
Validate Inputs and Handle Data Anomalies
Before executing WITH AS queries, validate the inputs and handle any potential data anomalies or inconsistencies. This includes validating user-provided parameters, checking for missing or incorrect data, and ensuring the data conforms to the expected format.
For example, if your query relies on user-provided parameters, validate the inputs to ensure they meet the required criteria. This can help prevent errors or unexpected behavior caused by invalid inputs.
Use Error Handling Constructs
Database management systems provide error-handling constructs such as TRY…CATCH blocks (in SQL Server) or exception handling mechanisms (in other databases). These constructs allow you to catch and handle errors that occur during query execution.
By wrapping your WITH AS queries within error handling constructs, you can gracefully handle exceptions, log error messages, and take appropriate actions to maintain data integrity and prevent query failures.
Implement Data Validation and Constraints
Another important aspect of error handling in WITH AS queries is implementing data validation and constraints. By enforcing constraints such as foreign key relationships, unique constraints, or check constraints, you can prevent data inconsistencies and ensure the integrity of your data.
For example, if your WITH AS query performs data modifications, such as inserting or updating records, ensure that the modified data adheres to the defined constraints. This can help prevent violations that could lead to errors or data corruption.
Log and Monitor Errors
Logging and monitoring errors in WITH AS queries is crucial for troubleshooting and identifying potential issues. Implement a robust logging mechanism that captures relevant error information, such as error messages, query details, and timestamps.
By monitoring error logs, you can identify recurring issues, track query performance, and proactively address potential problems. This can help in identifying patterns, optimizing query execution, and ensuring the overall reliability and stability of your WITH AS queries.
By following these error-handling best practices, you can ensure that your WITH AS queries are robust, reliable, and capable of handling unexpected scenarios. Proper error handling contributes to the overall stability and integrity of your database operations.
Real-World Examples and Use Cases of WITH AS in SQL
In this section, we will explore real-world examples and use cases of WITH AS in SQL. By examining practical scenarios where WITH AS can be applied, we can gain a better understanding of its versatility and its potential to solve complex data manipulation challenges. Let’s dive into some real-world examples.
Analyzing Sales Data with WITH AS for Monthly and Yearly Reports
Consider a scenario where you need to analyze sales data to generate monthly and yearly reports. WITH AS in SQL can be a valuable tool in such cases, allowing you to break down the complex query into smaller, more manageable parts.
Let’s take a look at an example:
sql
WITH monthly_sales AS (
SELECT EXTRACT(MONTH FROM sale_date) AS month,
EXTRACT(YEAR FROM sale_date) AS year,
SUM(sale_amount) AS total_sales
FROM sales
GROUP BY EXTRACT(MONTH FROM sale_date), EXTRACT(YEAR FROM sale_date)
),
yearly_sales AS (
SELECT year,
SUM(total_sales) AS total_sales
FROM monthly_sales
GROUP BY year
)
SELECT month, year, total_sales
FROM monthly_sales
ORDER BY year, month;
In this example, we first define the monthly_sales
CTE to calculate the total sales for each month and year using the EXTRACT
function to extract the month and year from the sale_date
column. We then group the data by month and year and calculate the sum of the sales.
Next, we define the yearly_sales
CTE to calculate the total sales for each year by summing the sales from the monthly_sales
CTE. Finally, in the main query, we select the month, year, and total sales from the monthly_sales
CTE, ordering the results by year and month.
By utilizing WITH AS in this scenario, we can break down the complex task of analyzing sales data into smaller, more manageable steps. This approach improves code readability, simplifies the logic, and enhances the overall efficiency of the query.
Managing Hierarchical Data Structures with WITH AS in SQL
Hierarchical data structures, such as organizational charts or bill of materials, are commonly encountered in various applications. WITH AS in SQL provides a powerful solution to manage and manipulate hierarchical data efficiently.
Let’s consider an example of an organizational chart:
sql
WITH RECURSIVE org_chart AS (
SELECT employee_id, employee_name, manager_id, 0 AS level
FROM employees
WHERE manager_id IS NULL
UNION ALL
SELECT e.employee_id, e.employee_name, e.manager_id, o.level + 1
FROM employees e
INNER JOIN org_chart o ON e.manager_id = o.employee_id
)
SELECT employee_id, employee_name, level
FROM org_chart
ORDER BY level, employee_name;
In this example, we define the org_chart
CTE as a recursive CTE to traverse the organizational hierarchy. The initial anchor member selects employees with no manager (top-level employees), and subsequent members are generated by joining the employees
table with the previous members based on the manager ID.
The CTE includes the employee ID, employee name, manager ID, and the level of the employee within the hierarchy. In the main query, we select the employee ID, employee name, and level from the org_chart
CTE, ordering the results by the level and employee name.
By leveraging WITH AS in this scenario, we can easily navigate and manipulate hierarchical data structures. This approach simplifies complex operations, such as retrieving all descendants of a node or determining the depth of the hierarchy, making it an invaluable tool for managing hierarchical data.
Recursive Queries for Organizational Chart and Bill of Materials
Recursive queries, made possible by WITH AS in SQL, are particularly useful for tasks involving self-referential tables, such as managing organizational charts or bills of materials. Recursive CTEs allow you to traverse and manipulate data in a recursive manner, simplifying complex operations.
Let’s consider an example of retrieving all the subordinates of a manager in an organizational chart:
sql
WITH RECURSIVE subordinates AS (
SELECT employee_id, employee_name
FROM employees
WHERE manager_id = :manager_id
UNION ALL
SELECT e.employee_id, e.employee_name
FROM employees e
INNER JOIN subordinates s ON e.manager_id = s.employee_id
)
SELECT employee_id, employee_name
FROM subordinates;
In this example, we define the subordinates
CTE as a recursive CTE to retrieve all the subordinates of a given manager. The initial anchor member selects employees directly reporting to the specified manager. The subsequent members are generated by joining the employees
table with the previous members based on the manager ID.
The CTE includes the employee ID and employee name. In the main query, we select the employee ID and employee name from the subordinates
CTE, providing a list of all the subordinates of the specified manager.
Similarly, recursive CTEs can also be used to manage the bill of materials, where a product can have components that are also products with their own components. You can recursively traverse the bill of materials hierarchy to retrieve the complete list of components for a given product.
The versatility of recursive queries enabled by WITH AS makes them indispensable for managing hierarchical data and complex relationships.
Performing Complex Data Transformations with WITH AS in SQL
Data transformations are a common requirement when working with databases. WITH AS in SQL can simplify complex data transformations by breaking them down into smaller, more manageable steps.
Let’s consider an example of transforming raw sales data into a customer-centric view:
sql
WITH customer_sales AS (
SELECT c.customer_id, c.customer_name, SUM(s.sale_amount) AS total_sales
FROM customers c
JOIN sales s ON c.customer_id = s.customer_id
GROUP BY c.customer_id, c.customer_name
)
SELECT customer_id, customer_name, total_sales
FROM customer_sales
ORDER BY total_sales DESC;
In this example, we define the customer_sales
CTE to transform the raw sales data into a customer-centric view. We join the customers
and sales
tables based on the customer ID, calculate the sum of the sale amounts for each customer, and group the data by customer ID and customer name.
The CTE includes the customer ID, customer name, and total sales. In the main query, we select the customer ID, customer name, and total sales from the customer_sales
CTE, ordering the results by the total sales in descending order.
By leveraging WITH AS in this scenario, we can perform complex data transformations in a structured and organized manner. This approach enhances code readability, simplifies the logic, and improves the overall efficiency of the query.
Enhancing Performance of Large and Complex Queries with WITH AS
WITH AS in SQL can also be used to enhance the performance of large and complex queries. By breaking down the query into smaller, more manageable parts, you can optimize individual components and improve overall query performance.
Consider a scenario where you need to perform a complex analysis involving multiple joins, subqueries, and aggregations. By using WITH AS to break down the query into smaller CTEs, you can optimize each CTE individually, apply appropriate indexing strategies, and leverage query optimization techniques.
By optimizing the performance of each CTE and ensuring proper indexing, you can significantly reduce the execution time of the overall query. Additionally, the modular structure provided by WITH AS allows for better maintainability and flexibility, making it easier to modify or expand the query as needed.
In complex scenarios where performance is critical, WITH AS can be a valuable tool to tackle large and complex queries efficiently.
Conclusion
In this comprehensive blog post, we have explored the various aspects of WITH AS in SQL and its immense potential to enhance the efficiency, readability, and flexibility of SQL queries. We started by understanding the syntax and usage of WITH AS, including its structure and the different types of Common Table Expressions (CTEs) such as simple CTEs, recursive CTEs, and materialized CTEs.
We then delved into the numerous benefits and advantages of using WITH AS in SQL. We discussed how WITH AS improves query readability and maintainability by breaking down complex queries into smaller, more manageable parts. We explored how it reduces code duplication and enhances query efficiency by enabling the reuse of temporary result sets. We also saw how WITH AS simplifies complex queries, enables recursive operations on hierarchical data, and facilitates data transformation and preparation for analysis.
Furthermore, we explored advanced techniques and best practices for using WITH AS in SQL. We discussed how to utilize WITH AS with joins, subqueries, and aggregations to solve complex data retrieval problems. We explored the importance of filtering and ordering within WITH AS queries, as well as techniques for optimizing the performance of WITH AS queries through understanding execution plans, employing indexing strategies, and analyzing query performance. We also covered error handling and exception cases when working with WITH AS queries.
Throughout the blog post, we provided real-world examples and use cases to demonstrate the practical applications of WITH AS in SQL. From analyzing sales data and managing hierarchical structures to performing complex data transformations and enhancing the performance of large and complex queries, WITH AS proved to be a versatile and indispensable tool.
In conclusion, WITH AS in SQL is a powerful feature that empowers data professionals to write cleaner, more efficient, and more maintainable SQL queries. By leveraging its capabilities, you can simplify complex queries, enhance query performance, handle hierarchical data structures, and perform advanced data transformations. WITH AS unlocks the true potential of SQL and enables you to tackle a wide range of data manipulation challenges.
So, why wait? Start exploring and experimenting with WITH AS in SQL to elevate your SQL querying skills and unlock new possibilities in data manipulation and analysis.