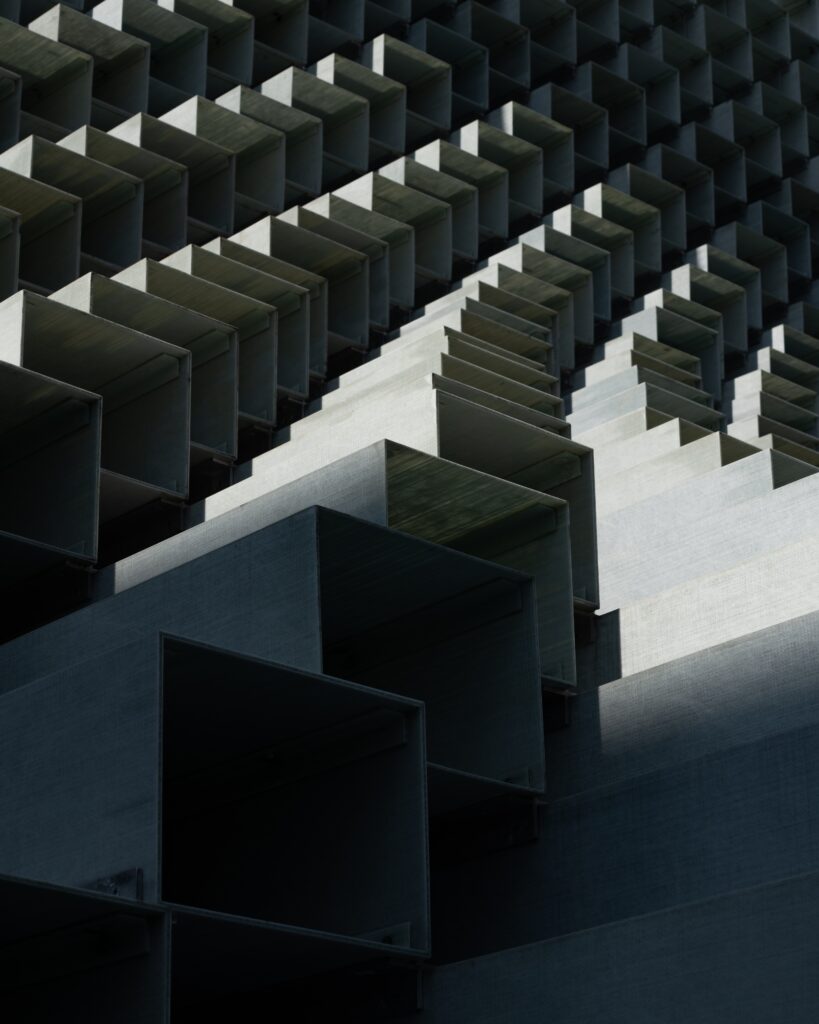
SQL (Structured Query Language) is a powerful tool for managing and manipulating data in relational databases. Whether you’re a data analyst, software developer, or database administrator, having a strong grasp of SQL query writing is essential for effectively interacting with databases and extracting valuable insights from data. In this comprehensive guide, we will dive deep into the world of SQL queries, exploring everything from the basics to advanced techniques, optimization, and best practices.
Introduction to Writing SQL Queries
In this section, we will lay the foundation for understanding SQL queries and their significance in database management. We will explore the definition of SQL, its importance in various industries, and common use cases that highlight the versatility of SQL query writing. Additionally, we will provide an overview of the SQL query structure, familiarizing ourselves with the essential clauses and keywords that form the basis of SQL queries.
What is SQL and why is it important?
SQL, or Structured Query Language, is a domain-specific language designed for managing and manipulating relational databases. It serves as the standard language for interacting with databases, enabling users to create, retrieve, update, and delete data. SQL plays a vital role in data-driven applications, acting as the bridge between software systems and databases.
The importance of SQL query writing cannot be overstated. Regardless of your role in the realm of data management, having a strong command of SQL is essential for efficient data retrieval, manipulation, and analysis. Whether you are a data analyst extracting insights from vast datasets, a software developer building applications with database integration, or a database administrator responsible for maintaining data integrity, SQL query writing is a fundamental skill that empowers you to interact effectively with databases and harness the power of data.
Benefits of mastering SQL query writing
Mastering the art of SQL query writing offers a multitude of benefits. By honing your SQL skills, you unlock the following advantages:
- Enhanced data retrieval: SQL queries provide a flexible and efficient way to retrieve data from one or multiple tables. Whether you need specific columns or wish to filter the data based on specific conditions, SQL allows you to extract the information you require, saving time and effort.
- Data manipulation and analysis: SQL empowers you to perform complex calculations, aggregate data, filter datasets based on specific criteria, and sort the results as needed. With SQL, you can transform raw data into meaningful insights, enabling informed decision-making and facilitating data-driven strategies.
- Seamless data integration: In today’s interconnected world, data often resides in multiple tables or even across different databases. SQL queries enable you to join tables, combine datasets, and analyze data from various sources. This facilitates comprehensive data analysis, reporting, and the ability to derive valuable insights from interconnected datasets.
- Efficient database management: SQL queries go beyond data retrieval and manipulation. They allow you to insert new data into tables, update existing records, and remove unnecessary data. By mastering SQL query writing, you gain the skills necessary for maintaining the integrity and consistency of your databases.
- Improved job prospects: In today’s data-driven world, proficiency in SQL query writing is highly sought after by employers across various industries. By acquiring this skill, you enhance your employability and open doors to a wide range of job opportunities in fields such as data analysis, database administration, business intelligence, and software development.
Common use cases for SQL queries
SQL queries find applications in numerous industries and scenarios. Let’s explore some of the common use cases where SQL query writing plays a pivotal role:
- Business intelligence and analytics: SQL is extensively used in business intelligence to extract, transform, and analyze data from databases. By leveraging SQL queries, analysts can uncover trends, patterns, and insights that drive strategic decision-making.
- E-commerce and online retail: SQL queries are crucial for managing product catalogs, tracking sales, and analyzing customer behavior. They enable retailers to gain a comprehensive understanding of customer preferences, optimize inventory management, and personalize the shopping experience.
- Healthcare and medical research: In the healthcare industry, SQL queries are employed to manage patient records, track medical data, and conduct research on health trends. SQL enables healthcare professionals to access critical information quickly and efficiently, leading to improved patient care and medical advancements.
- Financial services: SQL queries play a crucial role in financial services, facilitating tasks such as managing transactions, tracking account balances, and generating financial reports. The ability to retrieve and analyze financial data swiftly and accurately is essential in this industry.
- Web and mobile app development: Behind the scenes of every web or mobile application lies a database that stores and retrieves data. SQL queries are used to interact with databases, ensuring seamless data integration and efficient data retrieval for applications.
Overview of the SQL query structure
To effectively write SQL queries, it is essential to understand their structure and the various components that constitute them. A typical SQL query consists of several clauses and keywords:
- SELECT: This clause specifies the columns or expressions to retrieve in the result set. It allows you to choose the specific data you need from the database.
- FROM: The FROM clause identifies the table or tables from which to retrieve data. It provides the context for the query, indicating where the data resides.
- WHERE: The WHERE clause allows you to filter the data based on specific conditions. By specifying criteria, you can narrow down the result set to only include the desired records.
- GROUP BY: The GROUP BY clause is used to group the data based on specified columns for aggregation purposes. It facilitates the calculation of summary statistics or the application of aggregate functions.
- HAVING: The HAVING clause filters the grouped data based on specified conditions. It allows you to further refine the results obtained from the GROUP BY clause.
- ORDER BY: The ORDER BY clause sorts the result set based on specified columns or expressions. It enables you to organize the data in a specific order for better analysis and presentation.
- LIMIT: The LIMIT clause restricts the number of rows returned in the result set. It is particularly useful when dealing with large datasets and wanting to retrieve only a specific number of records.
Understanding the structure of SQL queries is crucial for constructing accurate and effective queries that meet your specific requirements. In the following sections, we will delve deeper into SQL query writing, starting with the basics and gradually progressing towards advanced techniques, optimization, and best practices. Ready to embark on this SQL journey? Let’s dive in!
Basic SQL Query Writing
In this section, we will explore the fundamentals of writing SQL queries. We will start by understanding how to select data from a single table and gradually progress to more advanced topics, such as joining tables to retrieve related data. By the end of this section, you will have a solid foundation in basic SQL query writing techniques.
Selecting data from a single table
The primary purpose of SQL queries is to retrieve data from databases. To accomplish this, we start with selecting data from a single table. This foundational concept allows us to extract specific columns, filter data based on conditions, sort the results, and limit the number of returned records.
Syntax for basic SELECT statements
The SELECT statement is the core of any SQL query. It allows us to specify the columns or expressions we want to retrieve from the database. The basic syntax for a SELECT statement is as follows:
sql
SELECT column1, column2, ...
FROM table_name;
Here, column1
, column2
, and so on represent the columns we want to include in the result set, while table_name
refers to the table from which we want to retrieve the data.
Retrieving specific columns
In many cases, we do not need to retrieve all columns from a table. SQL provides the flexibility to choose specific columns by listing them after the SELECT keyword. For example:
sql
SELECT column1, column2
FROM table_name;
By specifying only the necessary columns, we can optimize our queries and reduce the amount of data transferred from the database.
Filtering data using WHERE clause
To narrow down the result set, we can use the WHERE clause to apply filtering conditions. This allows us to retrieve only the rows that meet specific criteria. The WHERE clause follows the FROM clause in a SELECT statement and uses logical operators, comparison operators, and functions to define the conditions. For example:
sql
SELECT column1, column2
FROM table_name
WHERE condition;
The condition can be a simple comparison, such as column = value
, or a complex expression involving multiple conditions, such as column1 = value1 AND column2 > value2
.
Sorting data using ORDER BY clause
To organize the result set in a specific order, we can use the ORDER BY clause. This clause allows us to sort the rows based on one or more columns. By default, the sorting is done in ascending order, but we can specify the sorting order using the ASC (ascending) or DESC (descending) keywords. For example:
sql
SELECT column1, column2
FROM table_name
ORDER BY column1 ASC, column2 DESC;
In this example, the result set will be sorted in ascending order based on column1
and descending order based on column2
.
Limiting the number of results with LIMIT clause
In situations where we only need a specific number of rows from the result set, we can use the LIMIT clause. This clause allows us to restrict the number of rows returned by the query. For example:
sql
SELECT column1, column2
FROM table_name
LIMIT 10;
In this case, only the first 10 rows will be returned in the result set. The LIMIT clause is particularly useful when dealing with large datasets, as it allows us to retrieve a subset of records efficiently.
Joining tables to retrieve related data
In many database scenarios, data is distributed across multiple tables. To retrieve related data, we need to join these tables together based on common columns. SQL provides different types of joins, including inner joins, outer joins, left joins, and right joins, to facilitate this process.
Understanding different types of joins
- Inner join: An inner join returns only the rows that have matching values in both tables. It combines rows from two tables based on a matching column. If a row in one table has no matching row in the other table, it will not be included in the result set.
- Outer join: An outer join returns all rows from one table and the matching rows from the other table. If there is no match, the result will contain NULL values for the columns of the table with no match.
- Left join: A left join returns all rows from the left table and the matching rows from the right table. If there is no match, the result will contain NULL values for the columns of the right table.
- Right join: A right join is the opposite of a left join. It returns all rows from the right table and the matching rows from the left table. If there is no match, the result will contain NULL values for the columns of the left table.
Joining tables using JOIN clause
To perform a join operation, we use the JOIN clause in our SQL query. The JOIN clause specifies the tables to be joined and the join conditions. Here is an example of an inner join:
sql
SELECT column1, column2
FROM table1
INNER JOIN table2 ON table1.column = table2.column;
In this example, table1
and table2
are the tables being joined, and table1.column
and table2.column
represent the columns used for the join condition.
Applying join conditions
Join conditions specify how the tables should be joined. These conditions are typically based on the equality of values in specific columns. For example, to join tables based on a common user_id
column, we would use the following join condition:
sql
SELECT column1, column2
FROM table1
INNER JOIN table2 ON table1.user_id = table2.user_id;
By defining the join conditions, we can establish relationships between tables and retrieve related data.
Handling duplicate data with DISTINCT keyword
In some cases, joining tables may result in duplicate data. To eliminate these duplicates and retrieve only distinct values, we can use the DISTINCT
keyword. For example:
sql
SELECT DISTINCT column1, column2
FROM table1
INNER JOIN table2 ON table1.user_id = table2.user_id;
The DISTINCT
keyword ensures that only unique combinations of values are returned in the result set.
By understanding the basics of SQL query writing, including selecting data from a single table and joining tables to retrieve related data, you have taken the first steps towards mastering SQL. In the next section, we will explore advanced SQL query techniques, including aggregating data, using subqueries, and manipulating data with INSERT, UPDATE, and DELETE statements.
Advanced SQL Query Techniques
In this section, we will delve into advanced SQL query techniques that go beyond the basics. We will explore how to aggregate data using the GROUP BY and HAVING clauses, utilize subqueries and nested queries for complex data retrieval, and manipulate data using INSERT, UPDATE, and DELETE statements. By mastering these techniques, you will have a deeper understanding of SQL query writing and be able to tackle more complex data manipulation tasks.
Aggregating data with GROUP BY and HAVING
In many situations, we need to aggregate data to derive meaningful insights or perform calculations. SQL provides the GROUP BY clause and various aggregate functions to facilitate this process.
Grouping data using GROUP BY clause
The GROUP BY clause is used to group rows based on specified columns. It allows us to divide the result set into logical groups, which can then be used for aggregation. Here is an example:
sql
SELECT column1, aggregate_function(column2)
FROM table_name
GROUP BY column1;
In this example, column1
represents the column used for grouping, while aggregate_function
refers to one of the SQL aggregate functions, such as COUNT
, SUM
, AVG
, MIN
, or MAX
.
Applying aggregate functions
Aggregate functions perform calculations on a set of values and return a single value as the result. Common aggregate functions include:
- COUNT: Returns the number of rows in a group or the number of non-null values in a column.
- SUM: Calculates the sum of values in a group or a column.
- AVG: Computes the average of values in a group or a column.
- MIN: Finds the minimum value in a group or a column.
- MAX: Retrieves the maximum value in a group or a column.
By combining the GROUP BY clause with these aggregate functions, we can generate summary statistics and gain insights into our data.
Filtering grouped data with HAVING clause
The HAVING clause is used to filter the result set based on conditions applied to aggregated values. It works similarly to the WHERE clause but operates on the result of the GROUP BY and aggregate functions. Here is an example:
sql
SELECT column1, aggregate_function(column2)
FROM table_name
GROUP BY column1
HAVING condition;
The condition in the HAVING clause can involve comparisons, logical operators, and aggregate functions. It allows us to further refine the grouped data based on specific criteria.
Subqueries and nested queries
Subqueries, also known as nested queries, are queries embedded within another query. They allow us to break down complex problems into smaller, more manageable parts and retrieve data based on the results of inner queries.
Understanding subqueries and their purpose
A subquery is a query enclosed within parentheses and used as part of another query. It can be placed in the SELECT, FROM, WHERE, or HAVING clause of the outer query. The purpose of subqueries is to retrieve data based on values or conditions derived from the inner query.
Using subqueries in SELECT, WHERE, and FROM clauses
Subqueries can be used in various parts of an SQL query to achieve different results. For example, in the SELECT clause, we can use a subquery to retrieve a single value that represents a derived column:
sql
SELECT column1, (SELECT aggregate_function(column2) FROM table2) AS derived_column
FROM table1;
In this example, the subquery retrieves an aggregated value from table2
, which is then used as a derived column in the result set of the outer query.
Subqueries can also be employed in the WHERE clause to filter data based on the results of the inner query:
sql
SELECT column1
FROM table1
WHERE column2 IN (SELECT column2 FROM table2 WHERE condition);
In this case, the subquery retrieves a list of values from table2
based on a specific condition, and the outer query filters the result set based on those values.
Additionally, subqueries can be used in the FROM clause to create temporary tables that are used in the outer query:
sql
SELECT derived_table.column1
FROM (SELECT column1, column2 FROM table1) AS derived_table
WHERE condition;
In this example, the subquery creates a derived table that includes specific columns from table1
, and the outer query selects data from the derived table based on a condition.
Combining subqueries with other SQL operations
Subqueries can be combined with other SQL operations, such as joins and aggregate functions, to solve complex problems. For instance, we can use subqueries within a join condition to retrieve related data:
sql
SELECT column1, column2
FROM table1
INNER JOIN (
SELECT column3, column4
FROM table2
) AS subquery
ON table1.column1 = subquery.column3;
In this example, the subquery retrieves columns from table2
, and the outer query joins table1
with the result of the subquery based on the specified join condition.
By utilizing subqueries, we can break down complex problems into manageable components and retrieve data based on the results of inner queries.
Manipulating data with INSERT, UPDATE, and DELETE statements
While SQL queries are commonly associated with data retrieval, they also allow us to manipulate data in databases. We can insert new data, update existing records, and delete unnecessary data using the INSERT, UPDATE, and DELETE statements, respectively.
Inserting new data into tables
The INSERT statement is used to add new rows of data to a table. It allows us to specify the values for each column or insert data based on a query. Here is an example of inserting data with explicit values:
sql
INSERT INTO table_name (column1, column2)
VALUES (value1, value2);
In this example, table_name
refers to the table where we want to insert data, and column1
and column2
represent the columns into which we want to insert specific values.
Updating existing data
The UPDATE statement is used to modify existing data in a table. It allows us to change the values of specific columns based on certain conditions. Here is an example:
sql
UPDATE table_name
SET column1 = new_value1, column2 = new_value2
WHERE condition;
In this example, table_name
refers to the table where we want to update data, column1
and column2
represent the columns we want to modify, and new_value1
and new_value2
are the new values we want to assign.
Removing data from tables
The DELETE statement is used to remove rows from a table based on specific conditions. It allows us to delete data that is no longer needed or no longer meets certain criteria. Here is an example:
sql
DELETE FROM table_name
WHERE condition;
In this example, table_name
refers to the table from which we want to delete data, and condition
represents the criteria that determine which rows should be deleted.
Ensuring data integrity with transactions
When performing data manipulation operations, it is important to ensure data integrity. SQL provides the concept of transactions, which allows us to group multiple operations into a single unit of work. By using transactions, we can ensure that all the operations are executed together or rolled back if any operation fails.
In SQL, transactions are typically implemented using the BEGIN TRANSACTION
, COMMIT
, and ROLLBACK
statements. These statements allow us to start a transaction, commit the changes if all operations are successful, or roll back the changes if any operation fails.
By mastering the techniques of aggregating data with GROUP BY and HAVING clauses, utilizing subqueries and nested queries, and manipulating data using INSERT, UPDATE, and DELETE statements, you have taken a significant step towards becoming an expert in SQL query writing. In the next section, we will explore optimization and performance-tuning techniques to ensure that your SQL queries run efficiently and effectively.
Optimization and Performance Tuning
In this section, we will explore optimization techniques to improve the performance of SQL queries. We will discuss the importance of indexing and its impact on query execution, analyze query execution plans, identify and resolve performance bottlenecks, and optimize query performance through appropriate indexing and caching. By implementing these optimization strategies, you can ensure that your SQL queries run efficiently and deliver results in a timely manner.
Indexing and its impact on query performance
Indexing plays a crucial role in optimizing query performance. By creating indexes on columns used in search conditions or join operations, we can significantly improve the speed at which the database retrieves and filters data.
Understanding indexes and their types
An index is a data structure that allows the database to locate data quickly. It contains a copy of a subset of columns from a table, along with a pointer to the corresponding row. Indexes are created on one or more columns to facilitate efficient data retrieval.
There are different types of indexes, including:
- B-tree index: This is the most common type of index, suitable for most use cases. It organizes data in a balanced tree structure, enabling efficient data retrieval through binary searches.
- Hash index: This type of index uses a hash function to map the values of the indexed column to specific locations in the index structure. It is useful for equality searches but less effective for range queries.
- Bitmap index: A bitmap index uses a bitmap for each distinct value in the indexed column. It provides fast query performance for low cardinality columns or columns with few distinct values.
Creating and managing indexes
To create an index, we use the CREATE INDEX
statement. Here is an example:
sql
CREATE INDEX index_name ON table_name (column1, column2);
In this example, index_name
refers to the name of the index, table_name
represents the table on which the index is created, and column1
and column2
are the columns included in the index.
It is important to note that while indexes improve query performance, they also come with some overhead. Indexes consume disk space and require additional time for maintenance during data modification operations (such as inserts, updates, and deletes). Therefore, it is essential to strike a balance between the number of indexes and the performance benefits they provide.
Evaluating index usage and performance
To assess the effectiveness of indexes and identify areas for optimization, it is crucial to analyze the usage and performance of indexes. Database management systems (DBMS) provide tools and techniques to monitor index usage, such as examining query execution plans and using index statistics.
Query execution plans provide insights into how the database executes a specific query, including the indexes utilized, join methods employed, and filtering operations performed. By analyzing these plans, we can identify whether indexes are being utilized optimally or if there are potential areas for improvement.
Index statistics, such as the number of index scans, index seeks, and the ratio of seeks to scans, can also provide valuable information about index usage and performance. Monitoring and analyzing these statistics can help identify underutilized indexes, duplicate indexes, or indexes that need to be rebuilt.
Query optimization techniques
Query optimization involves improving the performance of SQL queries by identifying and resolving performance bottlenecks. By optimizing queries, we ensure that they execute efficiently and deliver results within an acceptable timeframe.
Analyzing query execution plans
Query execution plans are invaluable tools for understanding how the database processes a query. They provide insights into the steps taken by the database engine to retrieve and manipulate data. By analyzing these plans, we can identify potential performance issues, such as missing or unused indexes, inefficient join operations, or excessive data retrieval.
To obtain a query execution plan, most DBMS provide commands or tools specifically designed for this purpose. For example, in SQL Server, you can use the EXPLAIN
statement or the Query Execution Plan feature of SQL Server Management Studio.
Identifying and resolving performance bottlenecks
Performance bottlenecks can arise from various sources, such as inefficient query logic, lack of appropriate indexes, or inadequate hardware resources. To identify and address these bottlenecks, it is essential to perform thorough profiling and testing.
Profiling involves capturing and analyzing query execution data, such as query duration, CPU usage, and disk I/O. By profiling queries, we can identify the most time-consuming operations and focus our optimization efforts accordingly.
Testing involves executing queries under various scenarios and workloads to simulate real-world conditions. This helps identify performance issues that may not be apparent during development or testing with small datasets.
To resolve performance bottlenecks, we can employ various techniques, including:
- Rewriting queries: By modifying the query logic or restructuring the SQL statement, we can often improve performance. This may involve changing join order, eliminating unnecessary subqueries, or utilizing more efficient SQL constructs.
- Adding or modifying indexes: Through careful analysis of query execution plans and index statistics, we can identify opportunities to create or modify indexes to better suit query patterns. This can significantly enhance query performance.
- Optimizing database schema: The design of the database schema can impact query performance. By normalizing tables, denormalizing for performance-critical scenarios, or partitioning large tables, we can optimize data access and improve performance.
- Performance tuning hardware and infrastructure: In some cases, performance bottlenecks may be related to hardware limitations or inadequate infrastructure. Upgrading hardware, optimizing storage systems, or tuning database server configurations can help address these issues.
Caching and query result caching
Caching is a technique used to store frequently accessed data in memory for faster retrieval. By caching query results, we can eliminate the need for repetitive database access, resulting in significant performance improvements.
Query result caching involves storing the results of frequently executed queries in memory. When subsequent requests for the same query are received, the cached result is returned instead of executing the query against the database. This reduces the query execution time and reduces the load on the database server.
Many DBMS provide built-in caching mechanisms or caching frameworks that facilitate query result caching. By properly configuring and utilizing these caching mechanisms, we can improve the overall performance of our applications.
By implementing optimization and performance tuning techniques, including indexing, analyzing query execution plans, resolving performance bottlenecks, and utilizing caching, we can ensure that our SQL queries perform optimally. In the next section, we will explore best practices and tips for writing SQL queries that are clean, efficient, and secure.
Best Practices and Tips for Writing SQL Queries
Writing clean, efficient, and secure SQL queries is essential for effective database management. In this section, we will explore best practices and tips that will help you write SQL queries that are easy to understand, maintain, and optimize. We will cover topics such as writing clean and readable queries, handling NULL values and data inconsistencies, and ensuring security when writing SQL queries.
Writing clean and readable queries
Writing clean and readable queries is crucial for efficient database management and collaboration with other team members. Here are some best practices to follow:
Use proper indentation and formatting
Indentation and formatting play a vital role in making your queries more readable. Consistently indenting subqueries, clauses, and logical operators improves code structure and readability. Additionally, using line breaks, spacing, and aligning columns in the SELECT statement can enhance query legibility.
Choose meaningful table and column aliases
Using descriptive aliases for tables and columns can greatly improve query readability. Instead of using generic aliases like t1
, t2
, or c1
, c2
, opt for more meaningful aliases that reflect the purpose or content of the table or column.
Comment and document queries
Adding comments to your queries can provide valuable context and explanations for complex logic or unusual code patterns. Documenting queries can help other team members understand the purpose of the query and its expected behavior.
Handling NULL values and data inconsistencies
NULL values and data inconsistencies are common challenges when working with databases. Addressing these challenges in your queries ensures accurate and reliable results.
Dealing with NULL values in WHERE and SELECT clauses
When working with columns that may contain NULL values, it is essential to consider their impact on query results. Use appropriate filters such as IS NULL
or IS NOT NULL
to handle NULL values in the WHERE clause. In the SELECT clause, you can utilize functions like COALESCE
or IFNULL
to replace NULL values with specific values or expressions.
Avoiding data inconsistencies and ensuring data integrity
Data inconsistencies can lead to incorrect query results or unexpected behavior. To ensure data integrity, enforce proper constraints such as unique constraints, foreign key constraints, and check constraints. Regularly validate and clean your data to identify and correct any inconsistencies.
Security considerations when writing SQL queries
Security is a critical aspect of database management, and writing SQL queries with security in mind is essential to safeguard sensitive data and prevent unauthorized access.
Protecting against SQL injection attacks
SQL injection is a common security vulnerability that occurs when malicious code is injected into SQL statements through user input. To protect against SQL injection attacks, use parameterized queries or prepared statements instead of concatenating user input directly into the SQL statement. Parameterized queries ensure that user input is treated as data rather than executable code.
Implementing proper access controls and permissions
Granting appropriate access controls and permissions to database users is crucial for maintaining data security. Regularly review and update user access levels to ensure that each user has the necessary permissions required for their specific tasks. Follow the principle of least privilege, granting users only the permissions they need to perform their duties.
Encrypting sensitive data in the database
Sensitive data, such as passwords or personal information, should be stored securely in the database. Utilize encryption techniques, such as hashing and salting for passwords, to protect sensitive data from unauthorized access. Apply encryption at the column level or use transparent data encryption (TDE) for the entire database encryption.
By following these best practices and considering security considerations when writing SQL queries, you can ensure the integrity and confidentiality of your data.
Conclusion
In conclusion, understanding SQL queries and their significance in database management is essential in today’s data-driven world. SQL, or Structured Query Language, acts as the bridge between software systems and databases, enabling efficient data retrieval, manipulation, and analysis. Mastering SQL query writing offers numerous benefits, including enhanced data retrieval, data manipulation and analysis capabilities, seamless data integration, efficient database management, and improved job prospects in various industries.
SQL queries find applications in diverse fields, including business intelligence, e-commerce, healthcare, finance, and web and mobile app development. To effectively write SQL queries, it’s crucial to comprehend their structure, including essential clauses like SELECT, FROM, WHERE, GROUP BY, HAVING, ORDER BY, and LIMIT.
In the initial stages, we explored the fundamentals of SQL query writing, starting with selecting data from a single table and gradually progressing to more advanced topics like joining tables to retrieve related data. Understanding subqueries and nested queries also opened up possibilities for handling complex data retrieval scenarios.
The advanced SQL query techniques we discussed involved aggregating data with GROUP BY and HAVING clauses, manipulating data with INSERT, UPDATE, and DELETE statements, and ensuring data integrity with transactions.
Optimization and performance tuning techniques focused on the critical role of indexing in query performance, analyzing query execution plans, identifying and resolving bottlenecks, and optimizing performance through appropriate indexing and caching.
Lastly, we covered best practices for writing clean and readable queries, handling NULL values and data inconsistencies, and ensuring security when crafting SQL queries. These practices are essential to maintain data integrity, protect against security vulnerabilities, and facilitate collaboration among database professionals.
In your journey to becoming proficient in SQL query writing, incorporating these strategies and best practices will not only enhance your skills but also contribute to more efficient and secure database management.